class Node:
def __init__(self, value):
self.value = value
self.next = None
class LinkedList:
def __init__(self, value):
new_node = Node(value)
self.head = new_node
self.tail = new_node
self.length = 1
def print_list(self):
temp = self.head
while temp is not None:
print(temp.value)
temp = temp.next
def make_empty(self):
self.head = None
self.tail = None
self.length = 0
def append(self, value):
new_node = Node(value)
if self.head is None:
self.head = new_node
self.tail = new_node
else:
self.tail.next = new_node
self.tail = new_node
self.length += 1
my_linked_list = LinkedList(1)
my_linked_list.make_empty()
my_linked_list.append(1)
my_linked_list.append(2)
print('Head:', my_linked_list.head.value)
print('Tail:', my_linked_list.tail.value)
print('Length:', my_linked_list.length, '\n')
print('Linked List:')
my_linked_list.print_list()
"""
EXPECTED OUTPUT:
----------------
Head: 1
Tail: 2
Length: 2
Linked List:
1
2
"""
The provided Python code defines a simple linked list implementation with some basic functionalities. Let’s discuss each part of the code:
Class Definitions
- Node Class: This class represents a single node in the linked list. Each node has a
value
and anext
pointer, initially set toNone
. - LinkedList Class: This class represents the linked list itself. It has three attributes:
head
,tail
, andlength
.- Constructor (
__init__
): When a new LinkedList is created, it takes an initial value, creates a new node with this value, and sets both the head and tail to this node. The length is initialized to 1. print_list
Method: This method prints all the values in the linked list from the head to the tail.make_empty
Method: This method empties the linked list by setting the head and tail toNone
and resetting the length to 0.append
Method: This method adds a new node with the given value to the end of the linked list. If the list is empty (i.e., the head isNone
), it sets both the head and tail to the new node. Otherwise, it links the current tail to the new node and updates the tail. The length of the list is incremented by 1.
- Constructor (
Code Execution
- Initially, a
LinkedList
object (my_linked_list
) is created with the value1
. So, the list starts with one node containing the value1
. - The
make_empty
method is then called, which empties the linked list. - Two
append
calls are made, adding the values1
and2
to the list. - The final print statements display the values of the head, tail, and length of the list, as well as all the values in the linked list.
Expected Output
Based on the code’s logic, the expected output is correct:
Head: 1
Tail: 2
Length: 2
Linked List:
1
2
This output reflects that after creating the linked list, emptying it, and then appending two values, the list contains two nodes with values 1
and 2
, where 1
is the head and 2
is the tail of the list.
Summary
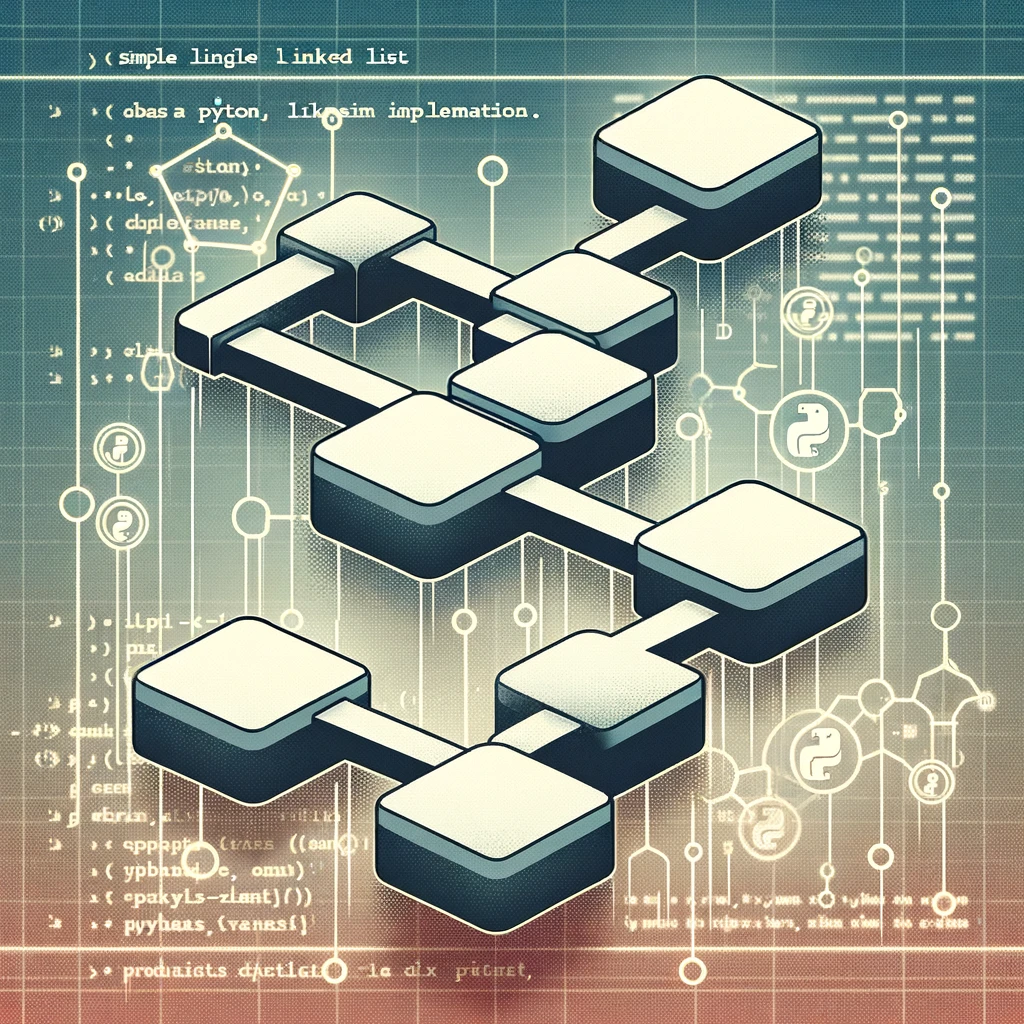
Article Name
A simple linked list implementation with some basic functionalities
Description
Explore the fundamentals of Python data structures with our guide to simple linked list implementation. This article delves into each aspect of linked list construction, from node creation to list manipulation methods like append and make_empty. Ideal for both beginners and seasoned programmers, this piece offers a clear understanding of basic linked list operations in Python, accompanied by practical code examples and explanations.
Author
techtinkerlabs
Publisher Name
TechTinkerLabs Media