Introduction to Python Linked Lists
Understanding the fundamental data structures is a key part of becoming proficient in Python, and the linked list is a critical piece of this puzzle. Python allows for the creation of these dynamic data structures through classes and constructors, providing a flexible way to store and manipulate collections of data.
Constructing the Linked List
To begin constructing a linked list in Python, we define a class LinkedList
and its constructor. The constructor is a special method that initializes the new instances of the class. In Python, constructors are defined using the __init__
method with self
as the first parameter, indicating that it pertains to the instance of the class.
class Node:
def __init__(self, value):
self.value = value
self.next = None
class LinkedList:
def __init__(self, value):
new_node = Node(value)
self.head = new_node
self.tail = new_node
self.length = 1
In the LinkedList
constructor, we start by creating a new node – an instance of the Node
class – which will serve as both the head and the tail of the list since it’s the only node in the list at the time of creation.
Expanding the Linked List
As our linked list grows, we will need methods to add new nodes. For this, we can create append
, prepend
, and insert
methods. Each of these methods will utilize the Node
class to create a new node that can be added to the list.
Working with the Linked List
Once the LinkedList
class is defined with its constructor, creating a new linked list is straightforward:
my_linked_list = LinkedList(4)
This line of code initializes a new linked list with a single node containing the value 4
.
Conclusion
The power of Python’s object-oriented programming shines in the way linked lists are implemented. By defining classes for the list and its nodes, Python programmers can build complex data structures capable of handling a variety of tasks.
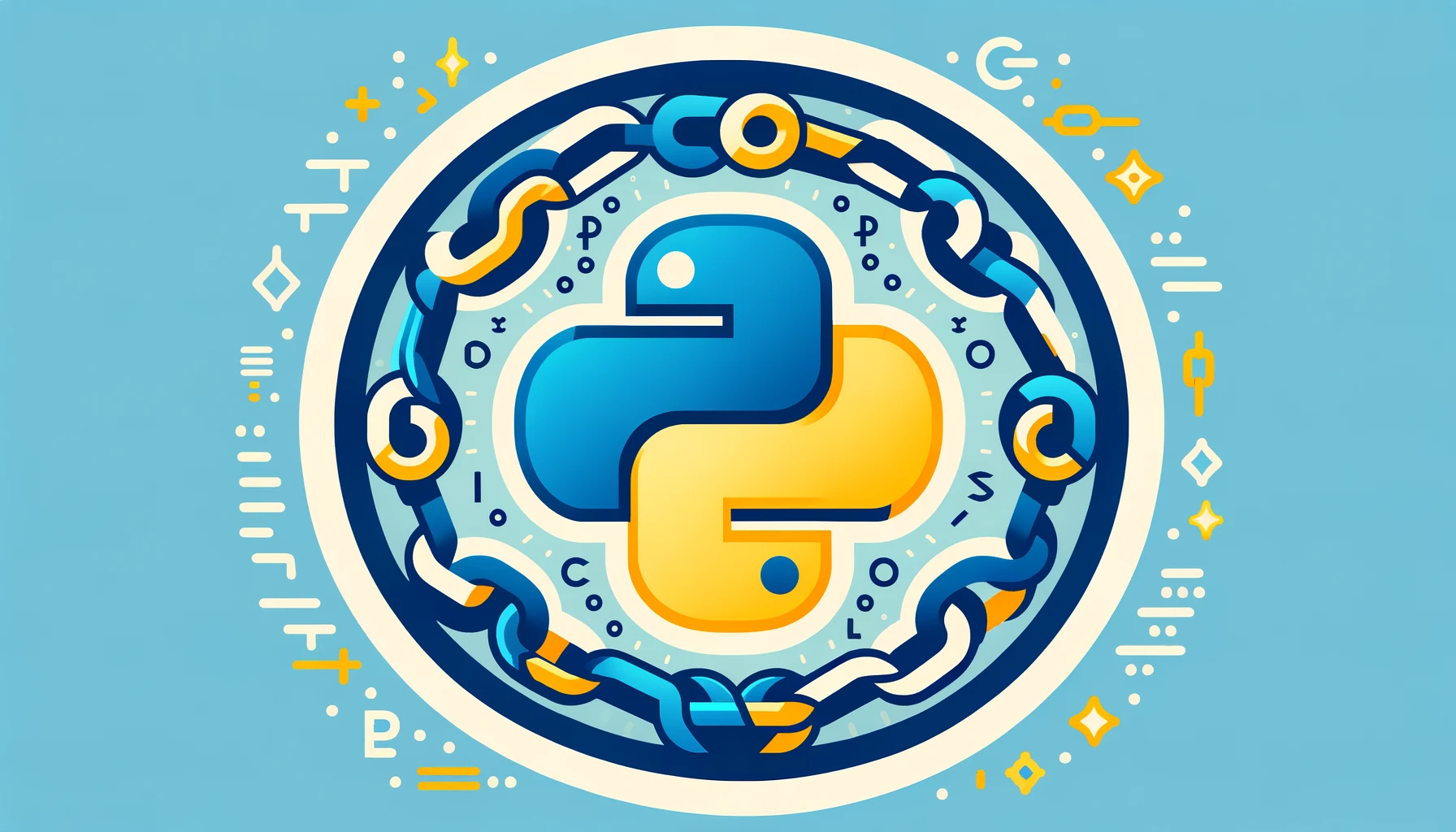