Introduction
This tutorial delves into the creation and management of a singly linked list in Python. A linked list is a fundamental data structure in computer science, ideal for dynamic data storage.
Step-by-Step Guide
Step 1: Define the Node Class
class Node:
def __init__(self, value):
self.value = value
self.next = None
Step 2: Create the LinkedList Class
class LinkedList:
def __init__(self, value):
new_node = Node(value)
self.head = new_node
self.tail = new_node
self.length = 1
def print_list(self):
temp = self.head
while temp is not None:
print(temp.value)
temp = temp.next
def make_empty(self):
self.head = None
self.tail = None
self.length = 0
def append(self, value):
new_node = Node(value)
if self.head is None:
self.head = new_node
self.tail = new_node
else:
self.tail.next = new_node
self.tail = new_node
self.length += 1
Step 3: Test the LinkedList
my_linked_list = LinkedList(1)
my_linked_list.make_empty()
my_linked_list.append(1)
my_linked_list.append(2)
print('Head:', my_linked_list.head.value)
print('Tail:', my_linked_list.tail.value)
print('Length:', my_linked_list.length, '\n')
my_linked_list.print_list()
Step 4: Analysis
To determine the output of the provided code, let’s analyze it step by step:
my_linked_list = LinkedList(1)
: This creates a newLinkedList
object with an initial value of 1. The list initially has one node (with value 1), and both the head and tail point to this node. The length is set to 1.my_linked_list.make_empty()
: This method sets thehead
andtail
of the list toNone
and resets thelength
to 0. The list is now empty.my_linked_list.append(1)
: Appends a new node with value 1 to the list. Since the list is empty, this new node becomes both the head and the tail of the list. The length of the list becomes 1.my_linked_list.append(2)
: Appends another node with value 2 to the list. This node is now the new tail of the list, while the head remains unchanged. The length of the list becomes 2.
Now, when the following print statements are executed:
print('Head:', my_linked_list.head.value)
: This will print the value of the head node, which is 1.print('Tail:', my_linked_list.tail.value)
: This will print the value of the tail node, which is 2.print('Length:', my_linked_list.length, '\n')
: This will print the length of the list, which is 2.my_linked_list.print_list()
: This will print the values of the nodes in the list, in this case, 1 and 2.
So, the expected output of the code is:
Head: 1
Tail: 2
Length: 2
1
2
Conclusion
This guide provides a foundational understanding of how to implement a basic singly linked list in Python, including essential methods for list management and traversal.
Summary
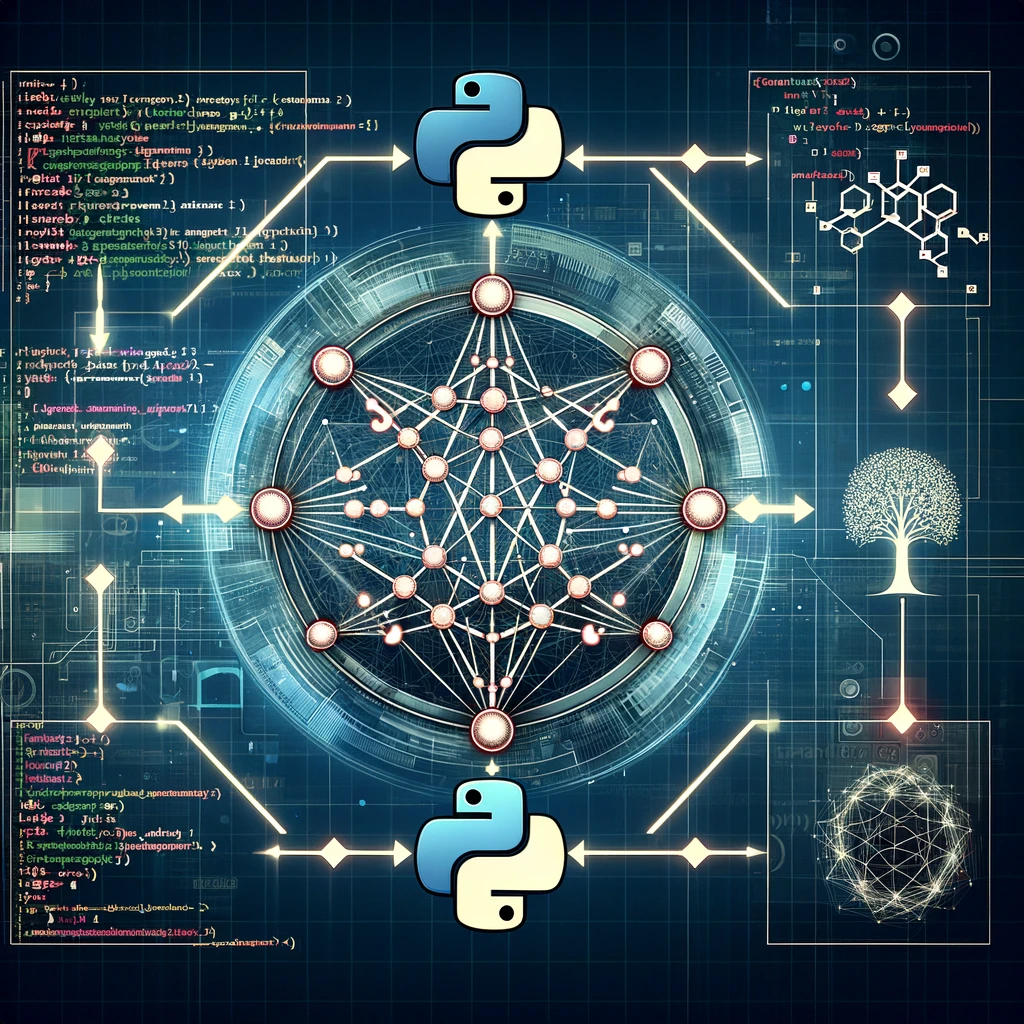
Article Name
Implementing a Singly Linked List in Python
Description
Explore the intricacies of implementing a singly linked list in Python with our comprehensive tutorial. Learn how to create, manage, and traverse linked lists through detailed explanations and code examples, perfect for budding programmers and seasoned coders alike.
Author
techtinkerlabs
Publisher Name
TechTinkerLabs Media