Introduction
In this tutorial, we will explore how to implement a print list method for linked lists in Python. This method is crucial for understanding and manipulating linked lists, a fundamental data structure in programming.
Implementation of Print List Method
To illustrate the concept, we’ll walk through a simple implementation of the print list method for a singly linked list. The linked list is composed of nodes where each node contains a value and a reference to the next node. Our goal is to traverse the list and print each value.
Step 1: Defining the Node Class
class Node:
def __init__(self, data):
self.data = data
self.next = None
Step 2: Creating the LinkedList Class
class LinkedList:
def __init__(self):
self.head = None
def print_list(self):
temp = self.head
while temp:
print(temp.data, end=" ")
temp = temp.next
Step 3: Using the Print List Method
# Creating an instance of LinkedList
llist = LinkedList()
llist.head = Node(11)
second = Node(3)
third = Node(23)
fourth = Node(7)
# Linking the nodes
llist.head.next = second
second.next = third
third.next = fourth
# Printing the list
llist.print_list()
Explanation
- Node Class: Defines the structure of a node in the linked list.
- LinkedList Class: Contains the
print_list
method. - print_list Method: Starts from the head of the list (
self.head
) and iterates through each node until it reaches the end (None
). It prints the value of each node during traversal.
Conclusion
Understanding and implementing the print list method provides a foundational understanding of linked lists in Python. This method is essential for visualizing and debugging linked list operations.
Summary
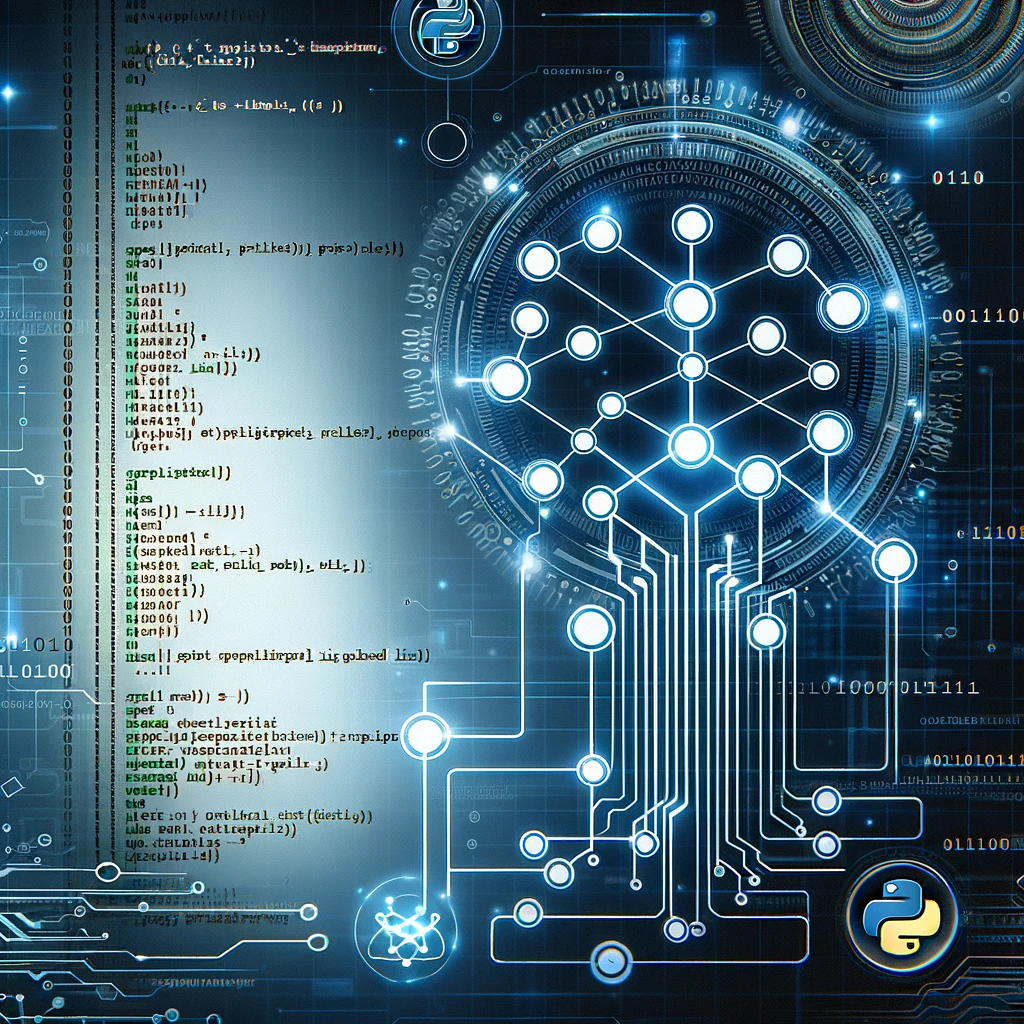
Article Name
Implementing and Understanding the Print List Method in Python
Description
Understanding and implementing the print list method provides a foundational understanding of linked lists in Python. This method is essential for visualizing and debugging linked list operations
Author
techtinkerlabs
Publisher Name
TechTinkerLabs Media