Introduction to Optimizing Algorithm Complexity
In computer science, Big O notation plays a critical role in optimizing algorithms for better performance. As developers, understanding and simplifying Big O notation can help us write more efficient code. In this article, we’ll explore the concept of ‘Drop Constants’ as a method to simplify Big O expressions.
The Concept of Drop Constants
‘Drop Constants’ is a principle used when evaluating the efficiency of algorithms. It’s based on the premise that in Big O notation, constants do not significantly affect the growth rate of an algorithm as the size of the input data increases. Let’s take a deeper look with a practical example.
Practical Example: Dual For-Loop in Python
Consider a Python function with two consecutive for loops, each iterating over ‘n’ elements:
def dual_for_loops(n):
for i in range(n):
print(i)
for j in range(n):
print(j)
# Run the function with n=10
dual_for_loops(10)
Running this function with an input of 10 prints out 20 items – two sequences from 0 through 9.
Interpreting the Output
When examining the output, we notice that the function prints each sequence once, resulting in a total of ‘2n’ outputs. You might be tempted to denote this as O(2n), but when simplifying using the ‘Drop Constants’ rule, we disregard the coefficient ‘2’. Thus, we represent the time complexity as O(n).
The Rule of Simplification: Drop Constants
The ‘Drop Constants’ rule helps us generalize the complexity of an algorithm. Whether the function runs 2n, 10n, or 100n times, the constant multiplier is dropped, and the time complexity is simplified to O(n). This simplification allows us to focus on the most significant factors that impact scalability and performance.
Conclusion
Understanding how to simplify Big O notation by dropping constants is a fundamental skill for software developers. It not only aids in writing clean and efficient code but also in communicating the performance characteristics of algorithms more effectively.
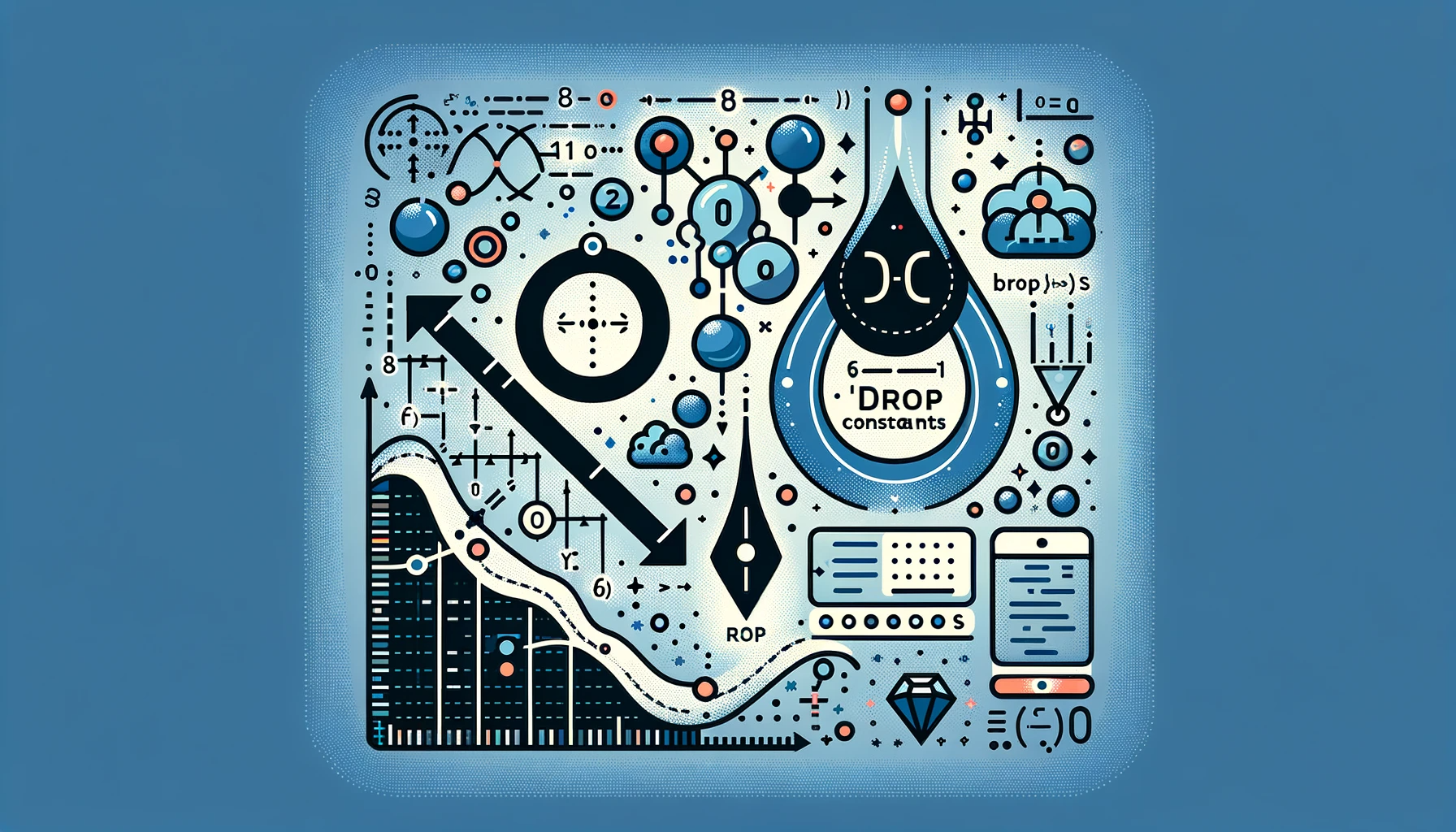