The Fundamentals of Big O Notation
Big O Notation is a mathematical concept used to describe the performance or complexity of an algorithm. Specifically, it measures how the runtime or space requirements of an algorithm grow as the input size grows. This fundamental concept in computer science helps developers understand the efficiency of their code and is crucial for optimizing applications, especially as data scales up.
Big O Complexity Classes
In an illustrative lecture, the importance of Big O Notation is discussed with clarity, highlighting the different classes of algorithm complexity:
- O(1) represents constant time complexity. No matter how large the input (n), the algorithm will execute in the same amount of time.
def check_first_element(list):
return True if list[0] == 1 else False
Below is an example of a Python function that demonstrates O(1), or constant time complexity:
def get_first_element(list):
"""
This function returns the first element of a given list.
The time complexity is O(1) because it performs a constant number of operations,
regardless of the size of the list.
"""
if list: # Check if the list is not empty
return list[0] # Return the first element
else:
return "List is empty" # Return a message if the list is empty
# Example usage:
my_list = [4, 2, 3, 5, 7]
print(get_first_element(my_list)) # Output will be 4, the first element of the list
No matter how many elements are in my_list
, get_first_element
performs only one action: it retrieves the first item. Therefore, the execution time does not change with the size of the input list, which is the defining characteristic of O(1) time complexity.
- O(log n) signifies logarithmic time complexity, often found in divide and conquer algorithms such as binary search.
def binary_search(array, target):
low = 0
high = len(array) - 1
while low <= high:
mid = (low + high) // 2
if array[mid] == target:
return mid
elif array[mid] < target:
low = mid + 1
else:
high = mid - 1
return None
Below is a sample Python code snippet that demonstrates a binary search algorithm, which is an example of O(log n)
time complexity. This algorithm divides the search interval in half with each step, reducing the number of elements to be searched and thus the time complexity logarithmically with the size of the input list.
def binary_search(arr, target):
"""
Perform a binary search of target in sorted array arr
:param arr: A list of elements sorted in ascending order
:param target: The value to search for
:return: The index of target in arr or -1 if not found
"""
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Example usage:
sorted_array = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
target_value = 7
# Should return the index of the target_value if it's present in the sorted_array
index = binary_search(sorted_array, target_value)
print(f"Index of the target value is: {index}")
In the example above, sorted_array
is a sorted list of integers, and target_value
is the number we want to find in sorted_array
. The binary_search
function will return the index of target_value
if it exists in the list or -1 if it does not. The binary search cuts the problem size in half with each iteration, hence it has a logarithmic time complexity O(log n)
.
- O(n) indicates linear time complexity, where the runtime grows directly in proportion to the input size.
def find_max_value(numbers):
max_value = numbers[0]
for number in numbers:
if number > max_value:
max_value = number
return max_value
Here is a sample Python code that demonstrates O(n) time complexity through a linear search algorithm. This function searches for a target value within an array by checking each element one by one.
def linear_search(arr, target):
"""
A simple linear search algorithm that runs in O(n) time complexity,
where 'n' is the number of elements in the array. It checks each
element one by one until the target is found or the end of the array is reached.
:param arr: List of elements to search through
:param target: The element to find in the array
:return: The index of the target element if found, otherwise -1
"""
for index, element in enumerate(arr):
if element == target:
return index # Target found
return -1 # Target not found
# Example usage:
# Searching for a value within an array of size 'n'
array = [3, 5, 2, 4, 9, 1]
target_value = 4
# The following function call runs in O(n) time complexity
result_index = linear_search(array, target_value)
result_index
In the example provided, the linear_search
function successfully found the target value 4 at index 3 in the array, demonstrating a linear relationship between the array size and the number of operations performed.
- O(n^2) is quadratic time complexity, often seen in algorithms with nested iterations, like bubble sort.
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
Below is an example implementation of the Bubble Sort algorithm in Python, which demonstrates O(n^2) time complexity due to its nested loops:
def bubble_sort(arr):
n = len(arr)
# Traverse through all array elements
for i in range(n):
# Last i elements are already in place
for j in range(0, n-i-1):
# Traverse the array from 0 to n-i-1
# Swap if the element found is greater than the next element
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Example usage:
my_list = [64, 34, 25, 12, 22, 11, 90]
sorted_list = bubble_sort(my_list)
print("Sorted array is:", sorted_list)
When this code is executed, it will sort the elements in my_list
in ascending order using the Bubble Sort algorithm. The sorting process is done by repeatedly stepping through the list, comparing adjacent elements, and swapping them if they are in the wrong order. This process is repeated until the list is sorted. Since there are two nested loops that each run up to n
times, the time complexity is O(n^2), where n
is the number of elements in the list.
Practical Implications of Big O
Understanding Big O is not just academic; it has real-world implications in software development, data analysis, and system design. Efficient algorithms are particularly crucial in fields such as data science, where large datasets are common.
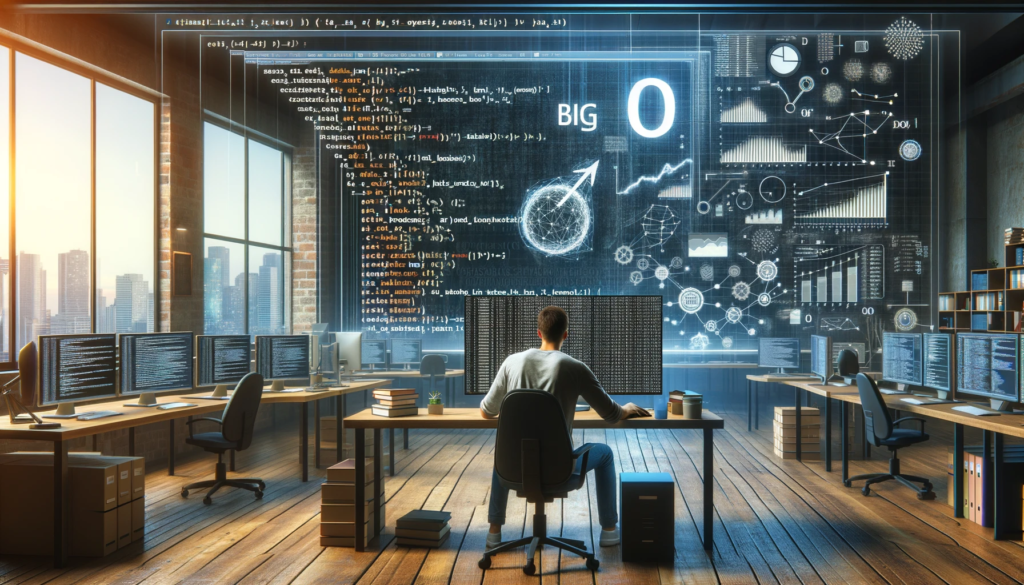
Conclusion
Big O Notation is an essential tool for any software developer or computer scientist. It provides a language to discuss algorithm efficiency objectively and to choose the right approach for a given problem.
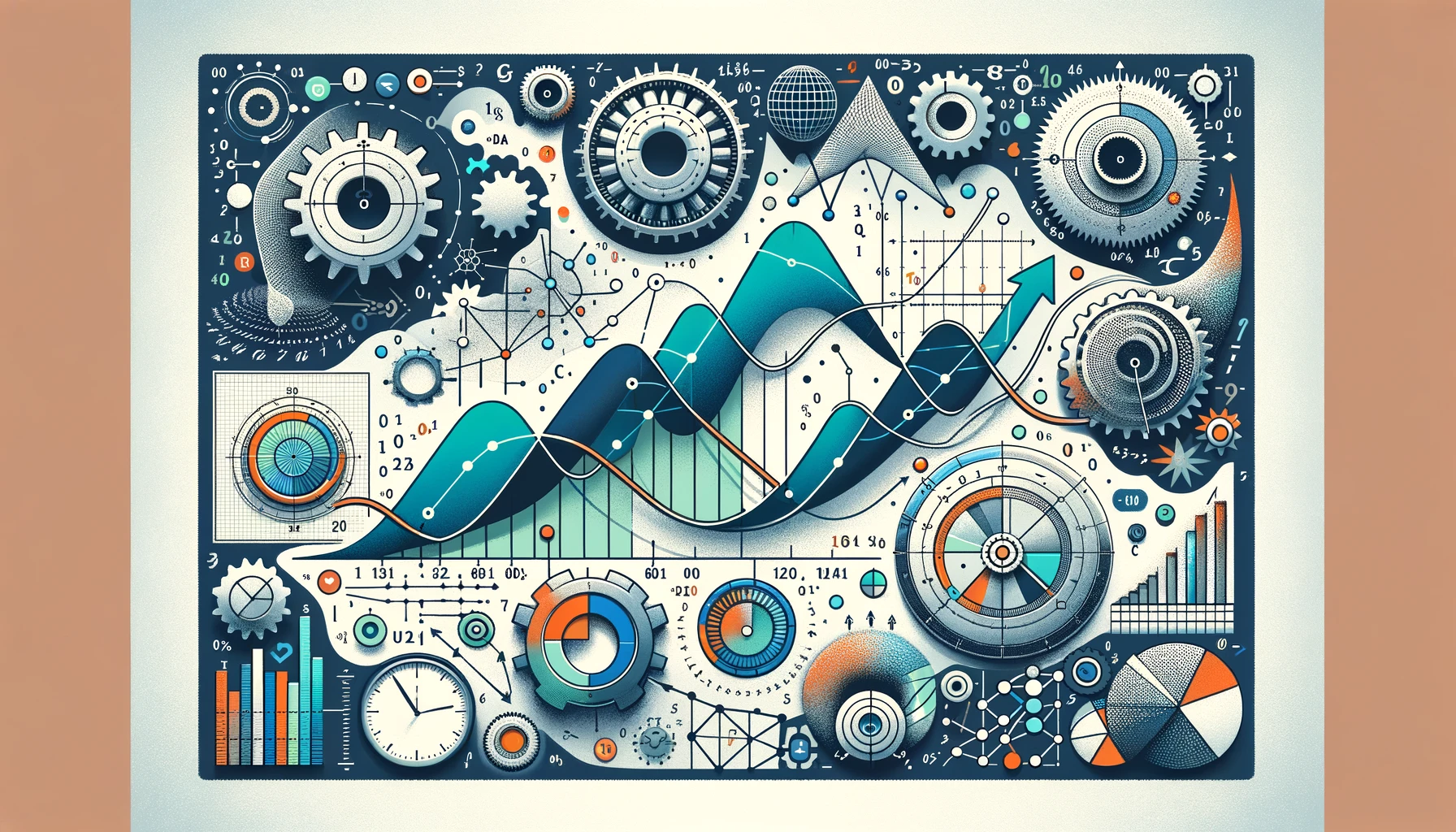