Decoding Complexity with Big O Notation in Algorithm Interviews
Big O notation is a mathematical concept that plays a pivotal role in computer science, especially during algorithm interviews. Often used to describe the efficiency of an algorithm, it can sometimes be the focus of tricky interview questions. Let’s explore a common interview scenario that involves Big O notation with multiple parameters.
The Gotcha Question: Dual Parameters in Big O
Imagine a simple scenario: a function that contains two consecutive for loops, each running ‘n’ times. At first glance, you might conclude that the complexity is O(2n), which simplifies to O(n) by dropping the constant. However, the plot twists when the function takes two different parameters, let’s say ‘a’ and ‘b’.
Example Code with Two Parameters:
def example_function(a, b):
for i in range(a): # This loop runs 'a' times
pass # Some operation
for j in range(b): # This loop runs 'b' times
pass # Some other operation
In this case, the first loop runs ‘a’ times and the second ‘b’ times. When calculating the Big O notation, you must consider each parameter individually. Thus, the time complexity for this function is O(a + b), not O(n).
Below is another example of a Python function that demonstrates the use of two parameters within the context of Big O notation. This function will contain two separate loops, each dependent on one of the parameters, a
and b
. The first loop performs an operation ‘a’ times, and the second loop performs a different operation ‘b’ times:
def process_two_parameters(a, b):
# Operation dependent on 'a'
for i in range(a):
print(f"Operation in the first loop, iteration {i}")
# Operation dependent on 'b'
for j in range(b):
print(f"Operation in the second loop, iteration {j}")
# Example usage:
process_two_parameters(5, 3)
When calling process_two_parameters(5, 3)
, the first loop will execute 5 times, and the second loop will execute 3 times. The Big O notation for this function is O(a + b), representing the total number of operations as a sum of the two separate loops. This is an essential concept in understanding how different parameters influence the complexity of an algorithm.
Here’s another example of a Python function that uses two different parameters to demonstrate a concept in Big O notation. This function features two distinct tasks, each with its own separate loop. The first loop runs ‘a’ times, and the second loop runs ‘b’ times, indicating that the time complexity is O(a + b) because the loops are not nested but consecutive:
def perform_separate_tasks(a, b):
# Task 1: Runs 'a' times
for i in range(a):
# Imagine some operation that takes constant time, like:
print(f"Performing task 1, iteration {i + 1}")
# Task 2: Runs 'b' times
for j in range(b):
# Another constant time operation, like:
print(f"Performing task 2, iteration {j + 1}")
# Example usage:
perform_separate_tasks(5, 10)
In the above code, if a
is 5, the first task will perform its operation 5 times. If b
is 10, the second task will perform its operation 10 times. Since the operations are not dependent on each other, the total number of operations is simply the sum of the two loops, hence O(a + b). This example is helpful for understanding how to analyze the time complexity of an algorithm with separate inputs.
Nested For Loops with Distinct Parameters:
def nested_loops(a, b):
for i in range(a): # Outer loop runs 'a' times
for j in range(b): # Inner loop runs 'b' times
pass # Some combined operation
For nested loops, the complexity is multiplied, leading to a complexity of O(a * b). This highlights the importance of differentiating between distinct input terms.
Here’s an example in Python that demonstrates nested for loops with distinct parameters, a
and b
. This structure results in a time complexity of O(a * b) because every iteration of the outer loop runs ‘a’ times and for each iteration of the outer loop, the inner loop runs ‘b’ times:
def nested_operations(a, b):
# Outer loop dependent on 'a'
for i in range(a):
# Inner loop dependent on 'b'
for j in range(b):
# Combined operation that occurs 'a' times for each 'b', resulting in 'a * b' operations total
print(f"Operation for pair ({i}, {j})")
# Example usage:
nested_operations(3, 4)
When you run nested_operations(3, 4)
, the outer loop will execute 3 times, and for each iteration of the outer loop, the inner loop will execute 4 times, resulting in a total of 12 prints to the console. This demonstrates that the total number of operations is the product of ‘a’ and ‘b’, which is indicative of the O(a * b) time complexity for nested loops with distinct parameters.
Here’s another example of nested for loops in Python, demonstrating the use of two distinct parameters a
and b
, which will result in a combined time complexity of O(a * b):
def calculate_combinations(a, b):
# Outer loop runs 'a' times
for i in range(a):
# Inner loop runs 'b' times for each iteration of the outer loop
for j in range(b):
# A hypothetical computation that would occur 'a * b' times in total
computation_result = i * j
print(f"Computation result for ({i}, {j}): {computation_result}")
# Example usage:
calculate_combinations(4, 5)
In this function, calculate_combinations
, the outer loop will execute a
times, and the inner loop will execute b
times for each iteration of the outer loop. If a
is 4 and b
is 5, the print statement will execute 20 times in total, which shows that the number of computations is the product of a
and b
, hence the time complexity is O(a * b). This code is often used to illustrate the basic principle of nested loop complexity in algorithm analysis.
The Importance of Precise Complexity Analysis
Understanding the correct use of Big O notation with multiple parameters is crucial. It provides a clear picture of an algorithm’s performance and ensures efficient code developmentâparticularly vital in fields like data science and machine learning.
Conclusion
Big O notation’s subtleties, such as dealing with multiple parameters, can make a significant difference in how we perceive an algorithm’s efficiency. Recognizing these nuances is key to excelling in coding interviews and developing efficient algorithms.
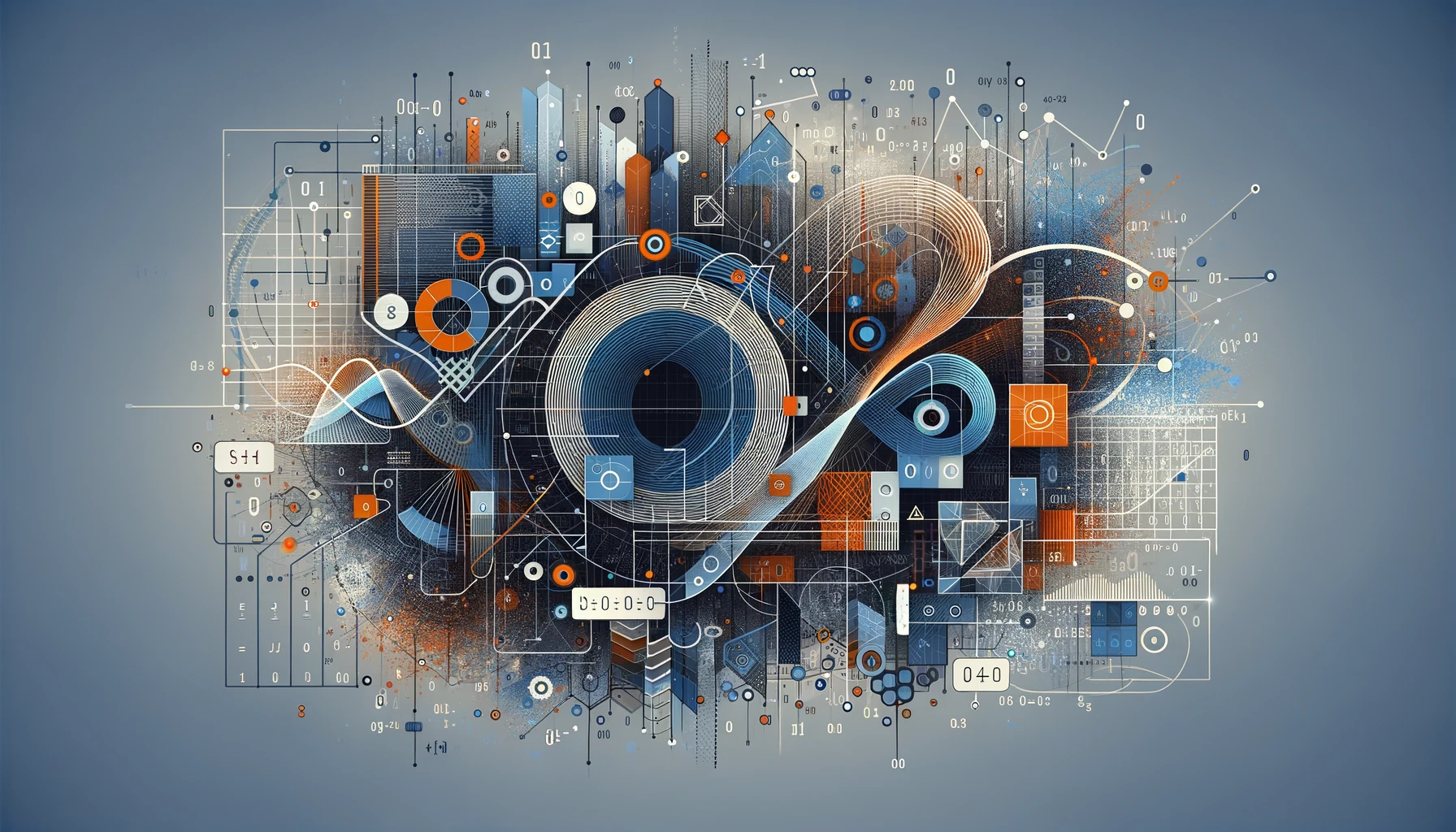