Introduction to Python Classes
In the realm of programming, classes form the bedrock upon which complex data structures are built. Classes in Python are akin to blueprints, detailing how to construct an object with specific attributes and behaviors. This introduction will provide insight into the essence of Python classes and how they are the foundation of every data structure we will encounter.
Python Classes: The Cookie Cutter Analogy
To understand classes, consider the analogy of a cookie cutter. Just as a cookie cutter shapes dough into cookies, a class shapes data into objects. In Python, when you create a class, you’re essentially creating your own custom data type, much like defining a new recipe for a cookie.
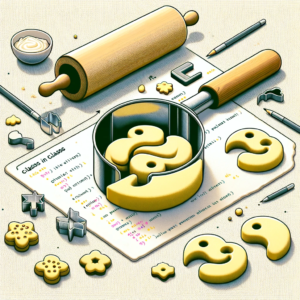
The Constructor: Bringing Classes to Life
The constructor in a class is the method that is called when a new object is created. It is defined using the def
keyword followed by __init__
, and it typically includes the self
parameter, which refers to the instance of the class. The constructor can take additional parameters, allowing for the initialization of each instance with specific attributes.
Here is a simple example of a Python class with a constructor that initializes the instance with specific attributes:
class Cookie:
def __init__(self, color, flavor):
self.color = color
self.flavor = flavor
# Creating an instance of the Cookie class
chocolate_chip_cookie = Cookie('golden brown', 'chocolate chip')
# Accessing the attributes of the chocolate_chip_cookie instance
print(f'The cookie is {chocolate_chip_cookie.color} and tastes like {chocolate_chip_cookie.flavor}.')
In this example, the Cookie
class has an __init__
method that is the constructor. This method takes two additional parameters besides self
: color
and flavor
. When a new Cookie
object is created, these parameters are used to initialize the color
and flavor
attributes of the object. The self
parameter refers to the current instance of the class, which allows you to access the attributes and methods associated with that instance.
Coding Example: Defining a Class in Python
Here’s a simple example of how a class can be defined and used in Python to create objects with different attributes:
class Cookie:
def __init__(self, color):
self.color = color
def get_color(self):
return self.color
def set_color(self, color):
self.color = color
# Creating instances of the Cookie class
cookie_one = Cookie('green')
cookie_two = Cookie('blue')
# Accessing and modifying the attributes of instances
print(cookie_one.get_color()) # Output: green
cookie_one.set_color('yellow')
print(cookie_one.get_color()) # Output: yellow
In this example, we define a Cookie
class with a constructor that sets the color of the cookie. We also define methods to get and set the color of a cookie.
From Cookies to Linked Lists
While our example uses a playful cookie analogy, the same principles are applied to more complex data structures like linked lists. A class for a linked list would have methods for appending, popping, and inserting elements, showcasing the versatility and power of classes in managing data.
# Let's redefine the classes and methods and run them to show the example usage as described.
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
if not self.head:
self.head = Node(data)
else:
current = self.head
while current.next:
current = current.next
current.next = Node(data)
def pop(self):
if self.head is None:
return None
elif self.head.next is None:
popped_data = self.head.data
self.head = None
return popped_data
else:
current = self.head
while current.next.next:
current = current.next
popped_data = current.next.data
current.next = None
return popped_data
def insert(self, index, data):
new_node = Node(data)
if index == 0:
new_node.next = self.head
self.head = new_node
else:
current = self.head
current_index = 0
while current is not None and current_index < index - 1:
current = current.next
current_index += 1
if current is None:
raise IndexError('Index out of bounds.')
new_node.next = current.next
current.next = new_node
def __str__(self):
elements = []
current = self.head
while current:
elements.append(current.data)
current = current.next
return ' -> '.join(str(e) for e in elements)
# Example usage of the LinkedList class
linked_list = LinkedList()
linked_list.append('Cookie 1')
linked_list.append('Cookie 2')
linked_list.insert(1, 'Cookie 1.5')
# Let's print the list and pop an element
output_before_pop = str(linked_list)
popped_cookie = linked_list.pop()
output_after_pop = str(linked_list)
(output_before_pop, f'Popped: {popped_cookie}', output_after_pop)
Result(‘Cookie 1 -> Cookie 1.5 -> Cookie 2’, ‘Popped: Cookie 2’, ‘Cookie 1 -> Cookie 1.5’)
Say, the above Python code for a simple linked list class has been executed. Here is how the linked list behaved during the example operations:
Initially, the linked list was:
Cookie 1 -> Cookie 1.5 -> Cookie 2
After popping an element (the last one, “Cookie 2”), the updated list became:
Cookie 1 -> Cookie 1.5
This illustrates how classes in Python can be used to create complex data structures like linked lists, with methods to append, insert, and pop elements.
Conclusion: The Significance of Python Classes
Classes are the cornerstone of object-oriented programming in Python. They allow programmers to encapsulate data and functionality together, creating reusable and organized code. As we dive deeper into Python, understanding classes becomes essential for mastering the language and implementing efficient data structures.
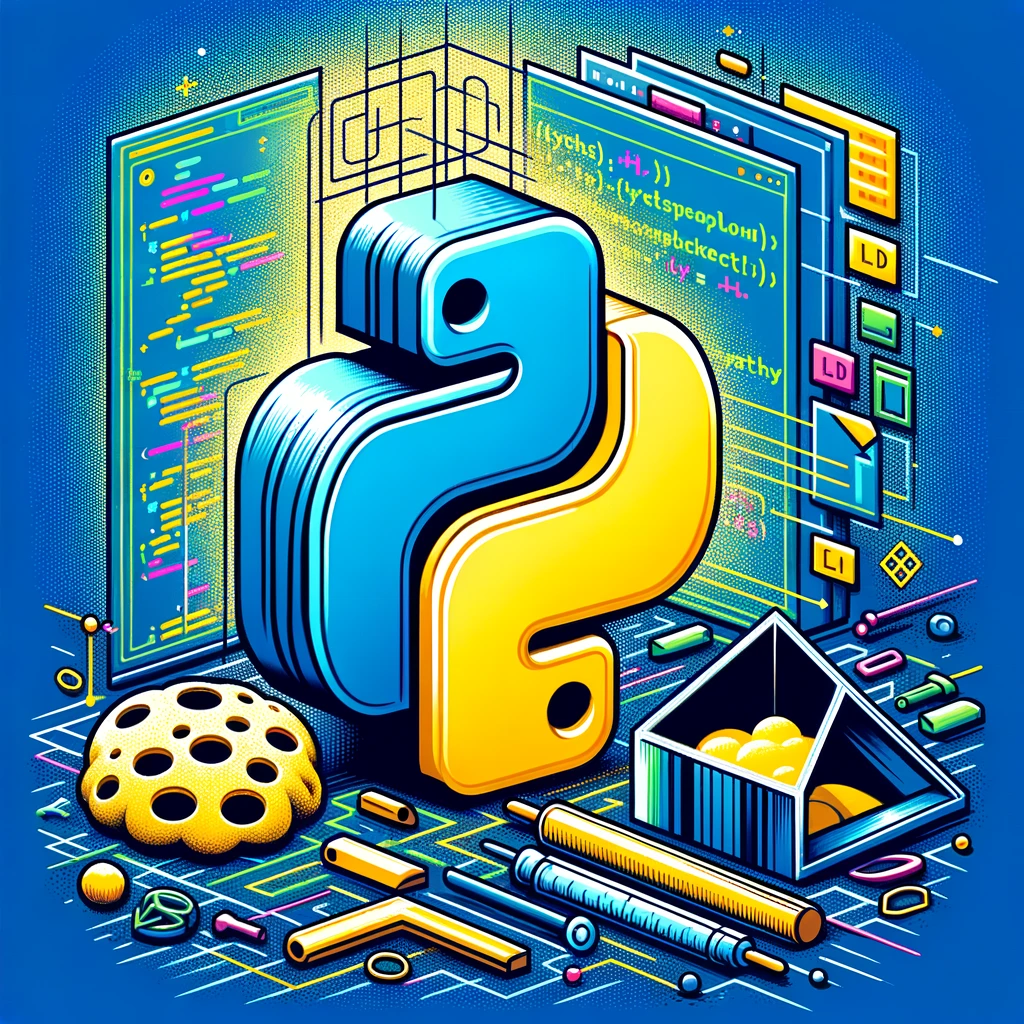