Introduction to Python Programming Pointers and Memory Allocation
Pointers are a fundamental concept in programming, essential for managing how memory is allocated and manipulated in various data structures. This article delves into how Python Programming pointers operate with different data types, using practical examples to demonstrate their function and importance in efficient code execution.
Pointers and Integers: Immutable Types
Consider two integer variables, num1
and num2
. When num1
is set to 11, it points to a memory location holding that value. If we then set num2 = num1
, num2
points to the same value as num1
. However, integers are immutable; when you change the value of num2
to 22, it now points to a different memory location, leaving the original 11 unchanged.
# Demonstrate integer immutability and memory allocation in Python
# Define two integer variables
num1 = 11
num2 = num1
# Display the values and the memory addresses
initial_num1_address = id(num1)
initial_num2_address = id(num2)
initial_values = (num1, num2)
initial_addresses = (initial_num1_address, initial_num2_address)
# Change the value of num2
num2 = 22
# Display the new values and memory addresses
new_num1_address = id(num1)
new_num2_address = id(num2)
new_values = (num1, num2)
new_addresses = (new_num1_address, new_num2_address)
(initial_values, initial_addresses, new_values, new_addresses)
Result((11, 11), (9793408, 9793408), (11, 22), (9793408, 9793760))
In the demonstration above, we have shown the behavior of integers in Python, which are immutable.
Initially, both num1
and num2
have the value 11, and they both point to the same memory location, as indicated by their identical memory addresses (9793408
).
After setting num2
to 22, the value of num1
remains unchanged at 11, while num2
changes to 22. Correspondingly, the memory address of num1
remains the same (9793408
), and num2
now points to a new memory location (9793760
).
This demonstrates that when the value of an immutable data type like an integer is changed, a new object is created at a different memory location.
Example Code: Pointers with Integers
# Initial values and their memory addresses
num1 = 11
num2 = num1
print("Initial values:")
print(f"num1: {num1}, Address of num1: {id(num1)}")
print(f"num2: {num2}, Address of num2: {id(num2)}")
# After changing num2
num2 = 22
print("\nAfter changing num2:")
print(f"num1: {num1}, Address of num1: {id(num1)}")
print(f"num2: {num2}, Address of num2: {id(num2)}")
Initial values:
num1: 11, Address of num1: 9793408
num2: 11, Address of num2: 9793408
After changing num2:
num1: 11, Address of num1: 9793408
num2: 22, Address of num2: 9793760
Pointers and Dictionaries: Mutable Types
Unlike integers, dictionaries are mutable. If dictionary1
points to a value of 11 and we set dictionary2 = dictionary1
, both point to the same dictionary. Changing dictionary2
‘s value to 22 also changes what dictionary1
is pointing to, illustrating the mutable nature of dictionaries.
Example Code: Pointers with Dictionaries
dictionary1 = {'value': 11}
dictionary2 = dictionary1
print(dictionary1, dictionary2)
print("dictionary1 address:", id(dictionary1), "dictionary2 address:", id(dictionary2))
dictionary2['value'] = 22 # Mutating the value
print("After mutation - ", dictionary1, dictionary2)
Pointers in Linked Lists and Garbage Collection
The concept of pointers becomes even more crucial when dealing with complex data structures like linked lists. When pointers are updated to refer to new nodes, the old ones may be left without a reference. Languages like Python handle this through garbage collection, automatically freeing memory that no longer has any variables pointing to it.
The Python code example below defines a Node
class, which will be the building block for our linked list, and a LinkedList
class that handles operations on the list. Initially, we create a simple linked list with three nodes containing the values 1, 2, and 3, respectively.
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
if not self.head:
self.head = Node(data)
else:
current = self.head
while current.next:
current = current.next
current.next = Node(data)
def print_list(self):
current = self.head
while current:
print(current.data, end=' -> ')
current = current.next
print('None')
# Create a linked list and add nodes to it
ll = LinkedList()
ll.append(1)
ll.append(2)
ll.append(3)
# Print the linked list
print("Initial linked list:")
ll.print_list()
# Update the pointer to refer to a new node, old node is left without a reference
ll.head.next = Node(4)
# Print the updated linked list
print("\nUpdated linked list after changing pointers:")
ll.print_list()
# At this point, the node containing '2' is no longer referenced and will be
# collected by Python's garbage collector eventually.
Initial linked list:
1 -> 2 -> 3 -> None
Updated linked list after changing pointers:
1 -> 4 -> None
After printing the initial linked list, we demonstrate updating pointers by changing the next
pointer of the head node to point to a new node containing the value 4. As a result, the node containing the value 2 is no longer reachable through the head of the list. In the output, we see that the linked list now consists of nodes with values 1 and 4, with the node containing the value 3 still reachable through the new second node. The node containing the value 2 is now unreferenced and will be cleaned up by Python’s garbage collection process since nothing points to it anymore.
The output of the code execution:
Initial linked list:
1 -> 2 -> 3 -> None
Updated linked list after changing pointers:
1 -> 4 -> None
This demonstrates the concept of pointers in a linked list and how Python’s garbage collector will automatically free memory from nodes that are no longer referenced. ​
Conclusion
Understanding pointers is vital for efficient memory management in programming. With the ability to manipulate memory locations, pointers are a powerful tool in a programmer’s arsenal, enabling dynamic data structures and efficient code.
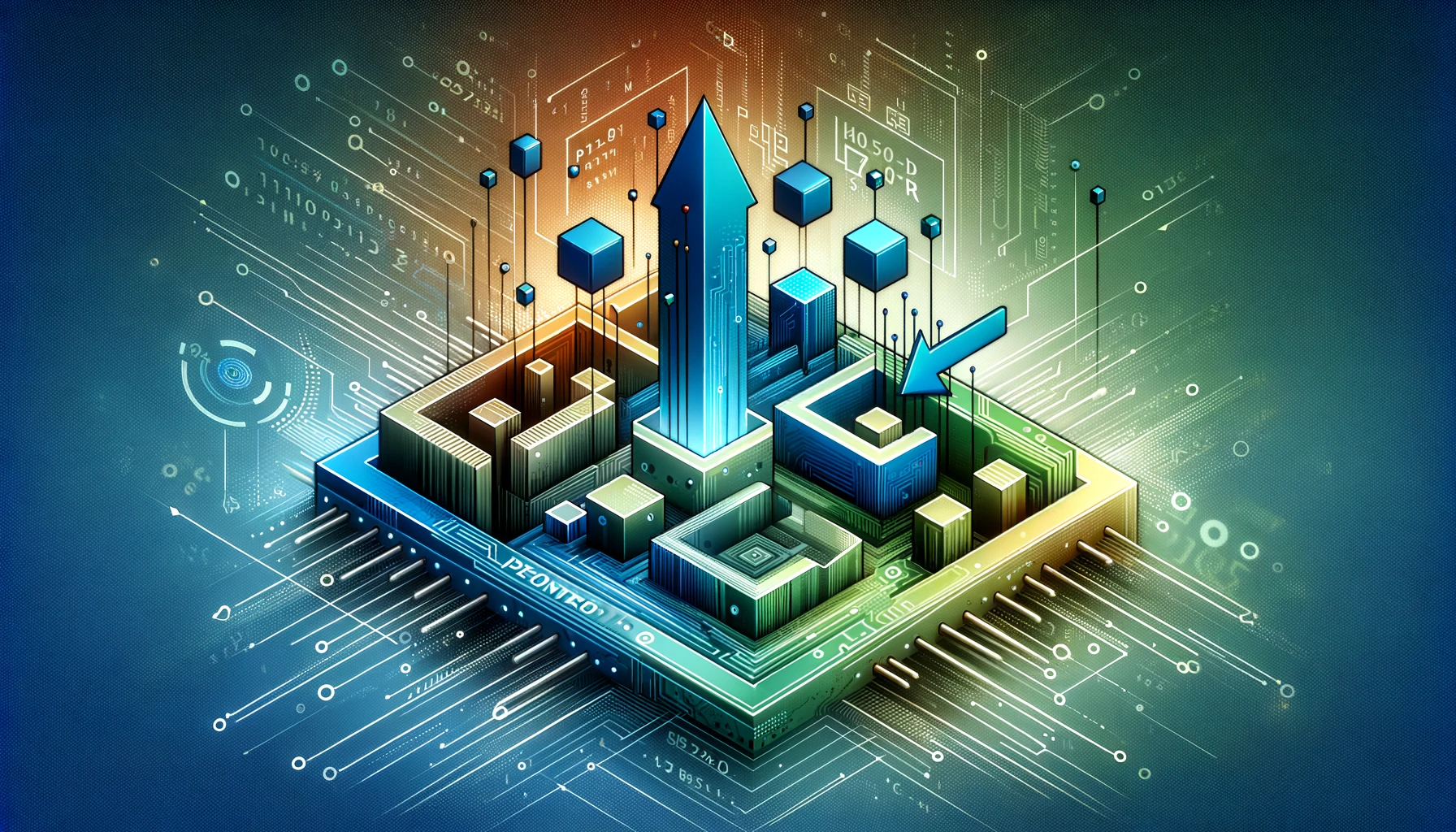