Exploring Computational Efficiency in Data Structures
In the realm of software development, understanding the Big O notation—particularly concerning lists—is fundamental. It is a concept that underpins performance considerations when choosing and implementing data structures in programming. Let’s explore the Big O of lists, a built-in data structure, and how it compares to others.
The Simplicity and Complexity of List Operations
Lists are versatile and commonly used in programming. They allow for straightforward operations like appending and popping elements without the need for re-indexing, which are O(1) operations—meaning they take constant time regardless of the list’s size. This simplicity is crucial when performance is a concern, especially for operations that are frequently used.
Here’s an explanation with an example to illustrate the concept of O(1) operations like appending and popping in lists to understand it clearly. Lists in programming are akin to dynamic arrays that can grow or shrink as needed. Two of the most common operations performed on lists are append
and pop
.
- Appending to a list means adding a new element to the end of the list.
- Popping from a list typically means removing the last element (though you can pop elements from any position).
Both these operations are considered O(1) operations. The ‘O’ stands for ‘Order of,’ and the ‘1’ signifies that the time it takes to perform these operations is constant. This means that no matter how large your list grows, appending a new item to the end or popping the last item will take the same amount of time.
Let’s see this with a Python example:
# Here's an empty list
my_list = []
# Appending items to the list
my_list.append('apple') # Appends 'apple' to the end of the list
my_list.append('banana') # Appends 'banana', list is now ['apple', 'banana']
# This operation is O(1) because it takes the same amount of time
# no matter how many items are already in the list.
# Popping the last item from the list
last_item = my_list.pop() # Removes and returns 'banana', list is now ['apple']
# Again, this pop operation is O(1) for the same reason.
# It takes a consistent amount of time regardless of the list's size.
In this example, regardless of whether the list has 10 items or 10,000 items, the time it takes to append
another item or pop
the last item does not increase. This predictability in performance is why O(1) operations are highly valued in software development, particularly when dealing with high-performance applications.
Append and Pop: The O(1) Operations
Consider a Python list with random numbers:
my_list = [11, 3, 23, 7]
Appending a new element is simple:
my_list.append(17) # This is O(1)
Popping the last element is equally straightforward:
my_list.pop() # This is O(1)
The Challenge with Insertions and Deletions
However, when you need to insert or delete an element at a specific index, especially at the beginning of the list, the complexity increases:
my_list.pop(0) # Removing the first item is O(n)
my_list.insert(0, 11) # Inserting at the beginning is also O(n)
This is because all subsequent elements need to be re-indexed, leading to an operation that scales with the list’s length, hence O(n).
Inserting in the Middle: Still O(n)
Even inserting in the middle of a list doesn’t reduce the complexity:
my_list.insert(1, "Hi") # This requires re-indexing the following elements, so it's O(n)
Search Operations: The Dichotomy
Searching by value is a linear operation:
# Searching for the value '7' will take O(n) as it may require checking each element
for i in my_list:
if i == 7:
break
Yet, accessing by index is constant time:
print(my_list[3]) # Accessing the fourth element is O(1)
Conclusion: Big O in Practice
In summary, when you’re working with lists, adding or removing elements at the end is highly efficient, while operations that involve the beginning or middle can be costly. Understanding these nuances is key to writing efficient, scalable code.
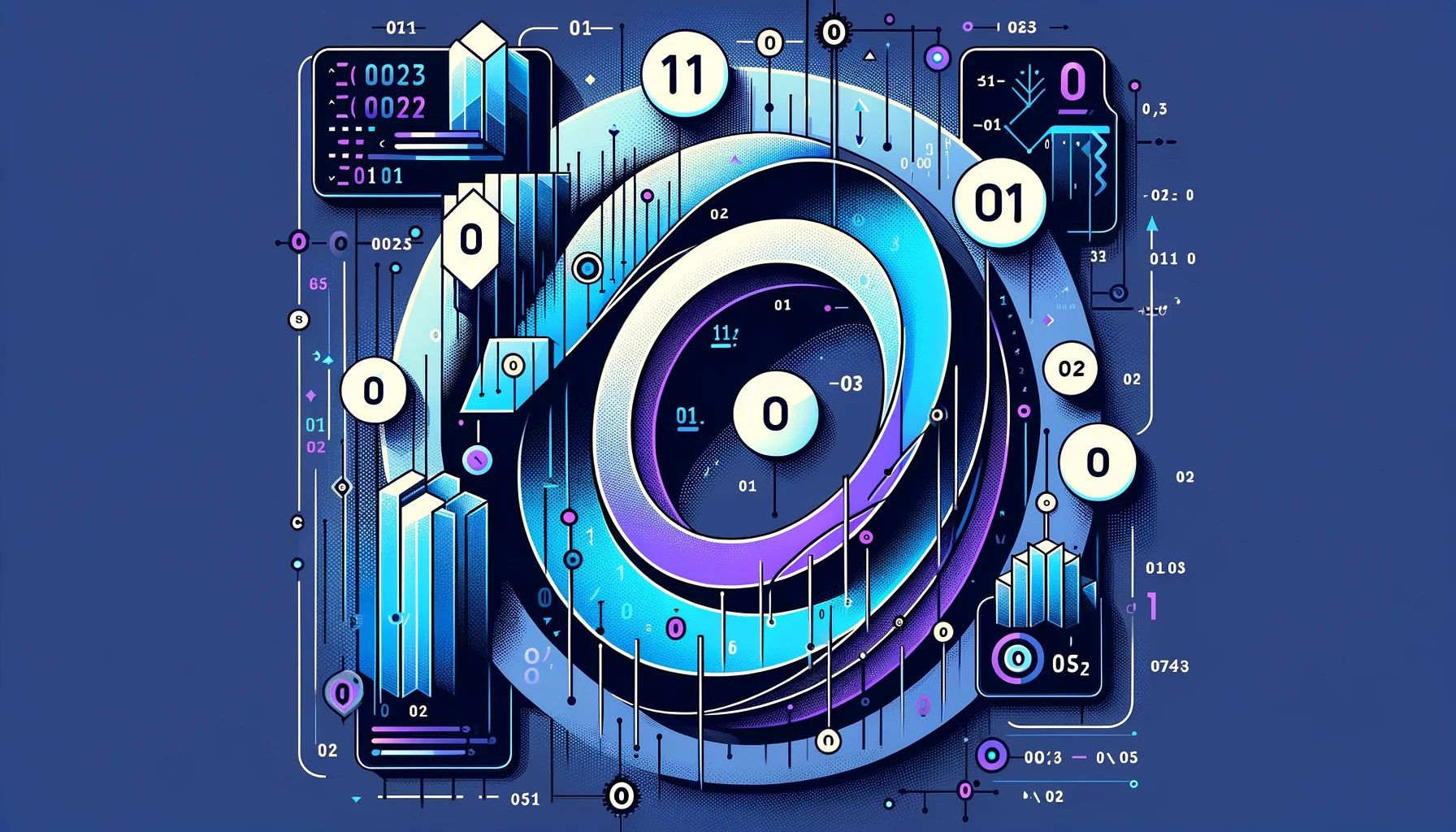